This is part two, go read part 1 first.
Last time we set up FreeDOS and installed DJGPP and RHIDE. Now let’s go through how to use them and write some simple programs.
I am going to assume you know how to use DOS. If not, all you need to know is how to make directories, move around the file system and how to copy files.
You also need to know DOS can only have filenames with 8 characters in total. Think carefully when naming files!
Where to store your code
I created a folder called dosdev
and inside that made folders for each of my programs. It doesn’t matter where you put them, just don’t call your main folder dev
for some reason.
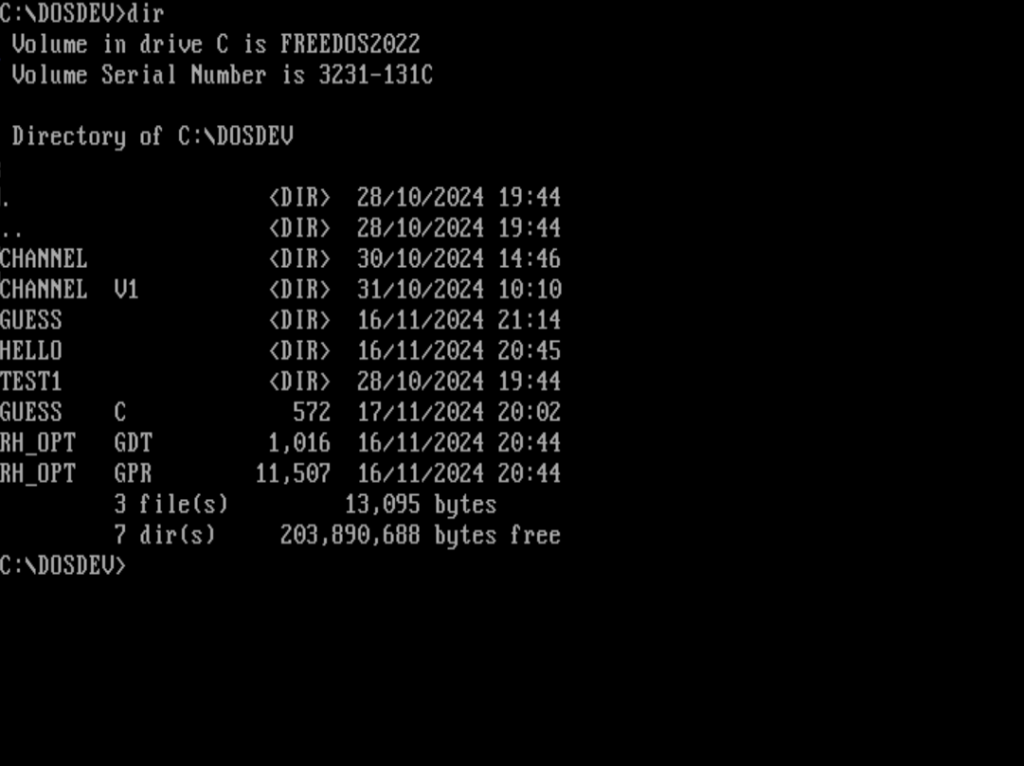
Hello World!
Yes, let’s make Hello World.
You need to make a folder for its code, go in there and launch rhide
by typing rhide
followed by a project name.
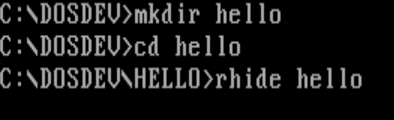
The main RHIDE window will appear. First we need to make a new source file and save it, even though it’s empty. Here’s a short video showing how.
Make the menus appear by pressing the alt
key on your keyboard.
Now we need to add this file to the project. Move to the Project Window by pressing the F6
key on your keyboard. Then press insert
to add the file using the file picker. Move between parts of the file picker (and other dialogs) using the Tab
key on your keyboard.
Weirdly you press Cancel
in the dialog to get out of it. Pressing OK
adds files. If you add a file by mistake, choose Delete
from the menu.
Now we just need to type in our code. Get back to the blue code window by pressing F6
on your keyboard again. Here is the code for the classic “Hello World” program in C.
#include <stdio.h>
void main()
{
printf ("Hello World!\n");
}
Code language: C++ (cpp)
This now needs compiling by choosing Run
. Press Alt
+R
then choose Run
from the menu. It will take a while to compile, and the output will flash on the screen. You can get it back by picking User Window
from the Windows
menu. Press Alt
+w
to get that menu open.
What is your name?
Here is the code for a slightly more complex program. To make use of it, try closing RHIDE and starting over in a brand new folder.
#include <stdio.h>
void main()
{
char name[50];
printf("What is your name?\n");
scanf("%s", &name);
printf("Hello %s\n", name);
char c = getchar();
}
Code language: C++ (cpp)
Number Guessing Game
And here’s a longer program that plays a simple number guessing game.
/*
Computer will think up a number
Repeat this until the game is over
Computer will ask player to make a guess
Player will type in their guess
Computer will work out if the guess is correct or not
The guess could be too high
The guess could be too low
The guess could be correct
The game is over
*/
#include <stdio.h>
#include <stdlib.h>
void main()
{
int secret = rand() % 100;
printf ("Please guess the number, it is between 1 and 100.\n");
while(1) {
printf ("Please type in your guess. >");
int guess = 0;
scanf ("%d", &guess);
if (guess > secret) {
printf ("Your guess is too big.\n");
} else if (guess < secret) {
printf ("Your guess is too small.\n");
} else {
printf ("Well done, you got it right!\n");
printf ("The number was %d\n", guess);
break;
} /* if */
} /* while */
printf ("Game over\n");
} /* main */
Code language: C++ (cpp)
Hopefully this has given you enough to get started. The Internet is full of examples and tutorials on how to write C, but it is mostly for modern systems. Next time I will show some more code that’s a bit more complex.
Until then, try to find some more simple examples and try them out to get used to RHIDE and the general process for editing code and fixing problems when they arise!
Leave a Reply